Data structures in Javascript as Arrays, Set, Map, Stack and Queue
A data structure is a useful form of data organization to use this data with ease.
The data structures available in Javascript are:
- Arrays or Lists. A set of data with different values that acquire a certain structure. The explanation is available at the following link about Arrays in Javascript.
- Set. A data set consisting of unique values or non-repeating values.
Its main available methods are: - add()→Add elements to the set
- has()→Check if an element exists inside the set
- delete()→Delete elements inside the set
- keys()→ Returns an array whose elements are strings from the applied Set or Array.
If we apply "keys" on a Set called "setExample" as follows, in the browser Console we can see the array as a whole in a drop-down menu:
console.log(Object.keys(setExample));
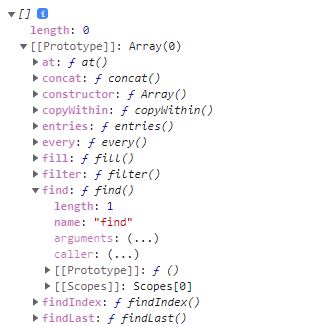
- values()→ Returns the value of an element of an array or set.
console.log(Object.values(setExample
)); - entries()→ Returns an array whose elements are arrays corresponding to the enumerable property
[key, value]
of Set or Array.
Example:
<script type="text/javascript">
const setExample = new Set([1, 2, 3, 5, 3, 2, 1]);
//It only collects the unique values that are 1, 2, 3, 5
console.log(setExample.has(3)); //true
setExample.add(2);
setExample.delete(1);
console.log(setExample);//{2, 3, 5}
console.log(Object.keys(setExample));
const newSet = {};
setExample.add(newSet);//Result: [];
</script>
- Map. It is a data structure that stores records as [key→value], where the key or value can be any data type.
Its main methods are:
get()→Get items
set()→Add elements
delete()→Delete elements
size()→Returns the size of the Map
Example:
<script type="text/javascript">
const mapExample = new Map();
mapExample.set('a', 1);
mapExample.set('b', 2);
mapExample.set('c', 4);
//Map stores data as key->value
console.log(mapExample.has('a'));//true
mapExample.set('c', 2);
console.log(mapExample.get('c'));// 2
mapExample.delete('b');//{'a' => 1, 'c' => 2}
console.log(mapExample);
const newMap = {};
mapExample.set(newMap, 3);
console.log(mapExample);// {'a' => 1, 'c' => 2, {…} => 3}
mapExample.delete(newMap);//{a: 1, c: 2}
</script>
- Queues and Stacks. They are abstract data structures that store values in a collection, whose utility is in the fact that these values can be added or extracted.
The difference between a queue and a stack is the order of fetching an element from the data structure.- Stack. Your data is stored in column form, that is, the data added last at the top of this column is the one that can be removed.
- Queue. The data is stored in the form of a column, adding the data in the initial part, and being able to withdraw the data only from the final part of the column.
In the following link about stacks and queues its operation is explained in more depth.
- Linked lists. It is a data structure where each item in the list has a reference to the next item, regardless of its order in memory or in a list itself. In the following link about Linked List its operation is explained in more depth.
Tips on SEO and Online Business